Azure landing zone implementation as an automated process using inputs from Low Level Design will reduce the overall build effort, bring in accuracy and mitigate the risk of duplication or other errors. Also this will help rebuild the environment from scratch with accuracy and minimal efforts.
The blog will help you create HUB and Spoke Network Azure using Low Level Design configuration details in Excel based LLD document. Using this example HUB and Spoke setup, you can plan to write similar code to add more functionalities to read required inputs from LLD.
A typical Azure HUB and Spoke looks like below
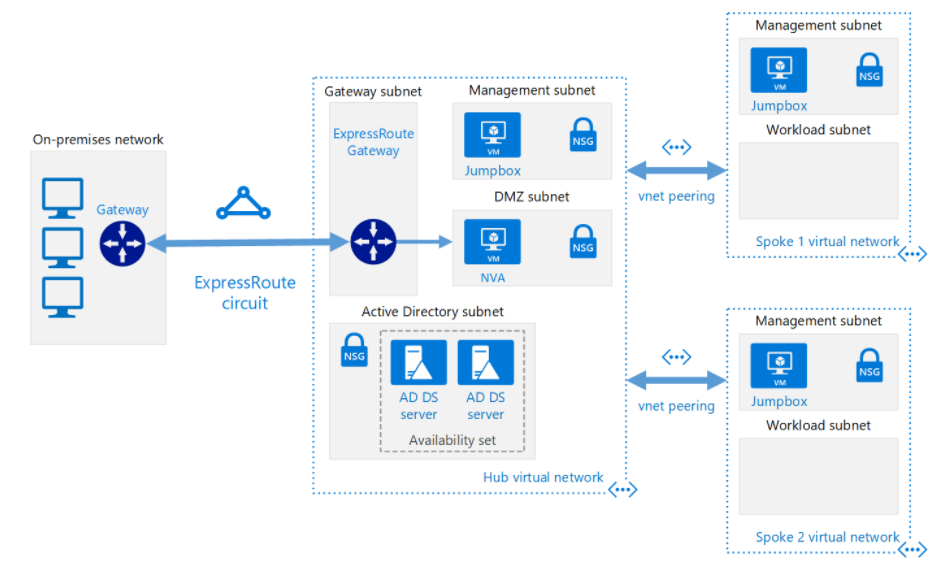
HUB – Centralized services ( Jumphost, AD VMs, AppGW, Virtual Network Gateway and Azure Firewall )
Spoke – Workload Infrastructure with three their architecture ( Web, App and DB )
Below sample excel document with Network Layout for HUB and Spoke
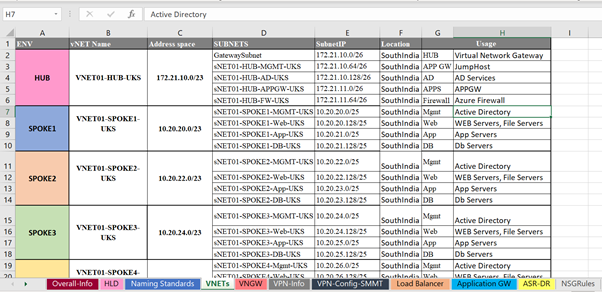
Use code from below onwards to setup the environment
————————————————————————————
#### Function to login to Azure Portal
Function LogintoAzure()
{
$AzureAccount = Connect-AzAccount
return $AzureAccount
}
####### Function for reading data inputs from LLD file ###################
function ReadNETXLS($xlspath, $xlsfilename, $SheetIndex, [int]$colmsno )
{
#$Error_workbookOpen
$filepath = “$xlspath” + “$xlsfilename”
$objExcel = New-Object -ComObject Excel.Application
$wb = $objExcel.workbooks.open(“$filepath”)
$cellarray = New-Object System.Collections.ArrayList
$WorkSheet = $wb.sheets.item([int]$SheetIndex)
$WorksheetRange = $workSheet.UsedRange
$RowNum = 0
$ColNum = 0
$ColCount = $WorksheetRange.Columns.Count – $colmsno
$RowCount = $WorksheetRange.Rows.Count
for ($i=2; $i -le $RowCount ; $i++){
for ( $j=2; $j -le $colCount; $j++ ){
$celldata=$WorkSheet.Cells.Item($i,$j).text
$cellinfo = $cellarray.Add($celldata)
}
}
$objExcel.Workbooks.Close()
return $cellarray
}
###### Function for setting up HUB and Spoke Infrastructure
Function SetupHUBSpoke($networkinfo)
{
$prevnet = “”
Foreach ($row in $networkinfo ) {
$arow=$row -split ‘,’
$vname = $arow[0]
$vaddr = $arow[1]
$sname = $arow[2]
$saddr = $arow[3]
$regn = $arow[4]
# If Virtual Network Name is same as Previously created Virtual Network Name
If ($vname -eq $prevnet) {
#Create subnets within same vNET once created”
$subnetConfig = Add-AzVirtualNetworkSubnetConfig `
-Name $sname `
-AddressPrefix $saddr `
-VirtualNetwork $vnetinfo
#Associate the subnet to the virtual network
$vnetinfo | Set-AzVirtualNetwork
$snetsuffix = $sname.Substring(0,6)
$nsgsuffix=$sname.Replace(“$snetsuffix”,”NSG”)
$nsgconfirm = Read-Host “Continue with creating default NSG rules for $sname ? [y/n]”
if ( $nsgconfirm -eq ‘y’ -or $nsgconfirm -eq ”){
$rdprule = New-AzNetworkSecurityRuleConfig -Name $nsgsuffix -Description “Allow RDP” `
-Access Allow -Protocol Tcp -Direction Inbound -Priority 3000 -SourceAddressPrefix `
* -SourcePortRange * -DestinationAddressPrefix * -DestinationPortRange 3389
$nsgrule = New-AzNetworkSecurityGroup -ResourceGroupName $rgname -Location $regn -Name `
“$nsgsuffix” -SecurityRules $rdprule
Set-AzVirtualNetworkSubnetConfig -Name $sname -VirtualNetwork $virtualNetwork -AddressPrefix $saddr -NetworkSecurityGroup $nsgrule
}
} Else {
# Else Create new Resource group, Virtual Network and first Subnet
$rgprefix=”RG-“
$rgsuffix=$vname.Replace(“VNET0″,”NET”)
$rgname = “$rgprefix$rgsuffix”
$addprefix = $vaddr.Trim(“`r?`n”)
#Create Azure Resource Group
$netrg= New-AzResourceGroup -Name $rgname -Location $regn
#Create vNET
$virtualNetwork = New-AzVirtualNetwork `
-ResourceGroupName $rgname `
-Location $regn `
-Name $vname `
-AddressPrefix $vaddr
#Create sNET
$subnetConfig = Add-AzVirtualNetworkSubnetConfig `
-Name $sname `
-AddressPrefix $saddr `
-VirtualNetwork $virtualNetwork
#Associate the subnet to the virtual network
$virtualNetwork | Set-AzVirtualNetwork
if ( $vname -like ‘*HUB*’ ){
$hubvnet = $vname
$hubnetwork = $virtualNetwork
}
else{
$snetsuffix = $sname.Substring(0,6)
$nsgsuffix=$sname.Replace(“$snetsuffix”,”NSG”)
$nsgconfirm = Read-Host “Continue with creating default NSG rules for $sname ? [y/n]”
if ( $nsgconfirm -eq ‘y’ -or $nsgconfirm -eq ”){
$rdprule = New-AzNetworkSecurityRuleConfig -Name $nsgsuffix -Description “Allow RDP” `
-Access Allow -Protocol Tcp -Direction Inbound -Priority 3000 -SourceAddressPrefix * -SourcePortRange * -DestinationAddressPrefix * -DestinationPortRange 3389
$nsgrule = New-AzNetworkSecurityGroup -ResourceGroupName $rgname -Location $regn -Name “$nsgsuffix” -SecurityRules $rdprule
Set-AzVirtualNetworkSubnetConfig -Name $sname -VirtualNetwork $virtualNetwork -AddressPrefix $saddr -NetworkSecurityGroup $nsgrule
}
# Setting up VNET PEERING between HUB and Spoke Network
$confirmation = Read-Host “Continue with creating vnet peering from $hubvnet to $vname ? [y/n]”
if ($confirmation -eq ‘y’ -or $confirmation -eq ”){
$peername1 = “$hubvnet”+”-TO-“+”$vname”
$peername2 = “$vname”+”-TO-“+”$hubvnet”
$peer1 = Add-AzVirtualNetworkPeering -Name $peername1 -VirtualNetwork $hubnetwork -RemoteVirtualNetworkId $virtualNetwork.Id
$peer2 = Add-AzVirtualNetworkPeering -Name $peername2 -VirtualNetwork $virtualNetwork -RemoteVirtualNetworkId $hubnetwork.Id
# Change the UseRemoteGateways property
$peer1.AllowGatewayTransit = $True
# Update the virtual network peering
Set-AzVirtualNetworkPeering -VirtualNetworkPeering $peer1
$peer2.UseRemoteGateways = $True
Set-AzVirtualNetworkPeering -VirtualNetworkPeering $peer2
}
}
}
$prevnet = $vname
$vnetinfo = $virtualNetwork
read-host “Press ENTER to continue…”
}
}
#Main Code
#
#############################################################################
#
Do
{
Write-Host “
———-MENU DXC Infrastructure Builds ———-
1 = Build HUB and Spoke setup
2 = Build Load Balancer
3 = Build Application Gateway
————————–“
$choice1 = read-host -prompt “Select number & press enter”
} until ($choice1 -eq “1” -or $choice1 -eq “2” -or $choice1 -eq “3” )
Switch ($choice1)
{
“1”
{
write “Building HUB and Spoke Network”
#############################################################################
##Setting Global Paramaters##
$ErrorActionPreference = “Stop”
$date = Get-Date -UFormat “%Y-%m-%d-%H-%M”
$workfolder = Split-Path $script:MyInvocation.MyCommand.Path
$logFile = $workfolder+’\’+$date+’.log’
Write-Output “Steps will be tracked on the log file : [ $logFile ]”
##Login to Azure##
$Description = “Connecting to Azure”
$Command = {LogintoAzure}
$AzureAccount = RunLog-Command -Description $Description -Command $Command -LogFile $LogFile
##Select the Subscription##
#
$Description = “Selecting the Subscription : $Subscription”
$Command = {Get-AzSubscription | Out-GridView -PassThru | Select-AzSubscription}
RunLog-Command -Description $Description -Command $Command -LogFile $LogFile
#############################################################################
#Set defaults
$netarray = ”
$netarray = New-Object System.Collections.ArrayList
#Reading inputs required for HUB and Spoke Network and adding to comma separated array
$xlspath = Read-Host “Enter the location of the PATH for input file”
$xlsfilename = Read-Host “Enter XLXS filename as inputs file”
$SheetIndex = Read-Host “Enter the cell number in the XLSX file”
$colmsno = Read-Host “Enter starting column of XLSX”
$data = ReadNETXLS $xlspath $xlsfilename $SheetIndex $colmsno
# Example below
#$data = ReadNETXLS ‘<PATH-TO-XLSS FILE’ ‘XLSX File NAME’ 4 2
$acount=$data.Length – 1
for ($k=0; $k -lt $acount ; $k++){
$vnetname = $data[$k]
$vnetaddress = $data[$k+1]
$snetname = $data[$k+2]
$snetaddress = $data[$k+3]
$netlocation = $data[$k+4]
$k = $k + 4
if ( $vnetaddress -eq ” -or $vnetname -eq ” ) {
$vnetaddress = $prevnetaddress
$vnetname = $prevnetname
}
$vnetaddress = $vnetaddress -replace “`n”, ” -replace “`r”,”
$vnetname = $vnetname -replace “`n”, ” -replace “`r”,”
$snetaddress = $snetaddress -replace “`n”, ” -replace “`r”,”
$snetname = $snetname -replace “`n”, ” -replace “`r”,”
$netlocation = $netlocation -replace “`n”, ” -replace “`r”,”
#SetupHUBSpoke Array with $vnetname $vnetaddress $snetname $snetaddress $netlocation
$netrow = $vnetname + “,” + $vnetaddress + “,” + $snetname + “,” + $snetaddress + “,” + $netlocation
$netinfo = $netarray.Add($netrow)
$prevnetaddress = $vnetaddress
$prevnetname = $vnetname
}
## Pass array to the function for creating HUB and Spokes
SetupHUBSpoke $netarray
#############################################################################
}
}